Overview
This project portfolio page serves to document my contributions to SocialCare, a software engineering project that was undertaken as part of the National University of Singapore (NUS) School of Computing’s beginner Software Engineering module, CS2103T. More information on this module can be found here.
SocialCare is an event and volunteer management system for administrators of social welfare organisations, which runs on desktops. The user interacts with it using the Command Line Interface (CLI), and it has a Graphical User Interface (GUI) created using JavaFX. The application is written in Java, and has about 10,000 lines of code.
Key Features of SocialCare:
-
Add, remove and store volunteers and events.
-
Assign volunteers to events.
-
Create event records for each volunteer consisting of the event and volunteer IDs, hours contributed and related remarks.
-
Export a PDF certificate detailing a volunteer’s involvement with the organisation (event records, contributed hours).
Through the use of the CLI, SocialCare enables the above functionalities to be executed faster than a typical mouse/GUI driven application.
Summary of contributions
This section summarizes my contributions to this project and to my fellow course mates throughout the course of the module. It includes enhancements made and a link to the code that I contributed as well.
-
Major enhancement: I added the ability to export volunteer certificates in PDF format.
-
What it does: This feature allows the user to export a volunteer’s event records and contributed hours in the form of a PDF certificate.
-
Justification: This feature improves the product significantly as it automates a task that is currently done manually by referencing files of volunteer records and painstakingly entering information into a word document. It also offers the user a way to access information from outside of the application as well (via portable and printable PDF files).
-
Highlights: This enhancement involved an in-depth analysis of available external open source libraries, and meticulous design of the PDF document to be exported.
-
Credits: This feature was developed with the use of Apache PDFBox, which provides the functionality to create and write to PDF files.
-
-
Minor enhancement: I added the total volunteer count to the status bar. This enhancement is in line with our application’s intention to provide the user with easy access to useful information pertaining to volunteers.
-
Code contributed: Here is a link to my code on the Project Code Dashboard.
-
Other contributions:
-
Documentation:
-
Revised the introduction sections of the README and Developer Guide (#109)
-
-
Community:
-
Reviewed PRs (with non-trivial review comments): #92, #107, #114, #232
-
Involved forum members in the resolution of a design consideration: #114
-
Shared a personal learning point with forum members: #143
-
Provided inspiration for the implementation of the import/export csv/xml feature, specifically through my handling of file export to main and backup filepaths in the
exportcert
feature I added.
-
-
Tools:
-
Integrated a third party library (Apache PDFBox) to the project (#161).
-
-
Project conceptualisation:
-
Set up and managed a shared Brainstorming Canvas to brainstorm on product morphing ideas using the 'Target User Profile-Problem-Value Proposition' framework.
-
Conceptualised and validated the idea for SocialCare through conversing with staff from 2 social welfare organisations that I have worked with in the past, who are part of our intended target group.
-
Contacted a software engineer from the industry to find out how Extreme Programming is actually employed, and shared the takeaways with my group and classmates.
-
-
Contributions to the User Guide
Given below is the section I contributed to the User Guide pertaining to the feature that I implemented, |
Exporting volunteer certificate : exportcert
Exports a PDF document to the user’s current working directory detailing a volunteer’s involvement with the organisation. This document includes:
-
Title: 'Certificate of Recognition'
-
Date of export
-
Volunteer name
-
Volunteer NRIC
-
List of events involved in - Event name, event ID, hours contributed, event start date and event end date
-
Total hours contributed across all events
Format: exportcert VOLUNTEER_INDEX
-
Exports a PDF certificate for the volunteer at the specified
VOLUNTEER INDEX
-
VOLUNTEER INDEX
must be a positive integer 1, 2, 3, … -
If the index given exceeds the number of volunteers in the displayed volunteer list, the message 'The volunteer index provided is invalid.' will be shown.
A certificate will be exported only if the volunteer has event records and at least 1 record has a positive, non-zero hour value. |
Example:
-
exportcert 1
Exports a PDF certificate for the volunteer at index 1 to the user’s current working directory. A success message will also be displayed in the following form: 'Certificate exported for volunteer at INDEX 1 to <EXPORT PATH>'.
Where will my files be exported to? If SocialCare has the permission to create folders on your machine, the certificates will be exported to a folder named 'Certs'. Else, they can be found next to the .jar file used to run the application. |
Here is what the exported certificate will look like: (Note that the number within the square brackets [ ] following the event name corresponds to the event ID)
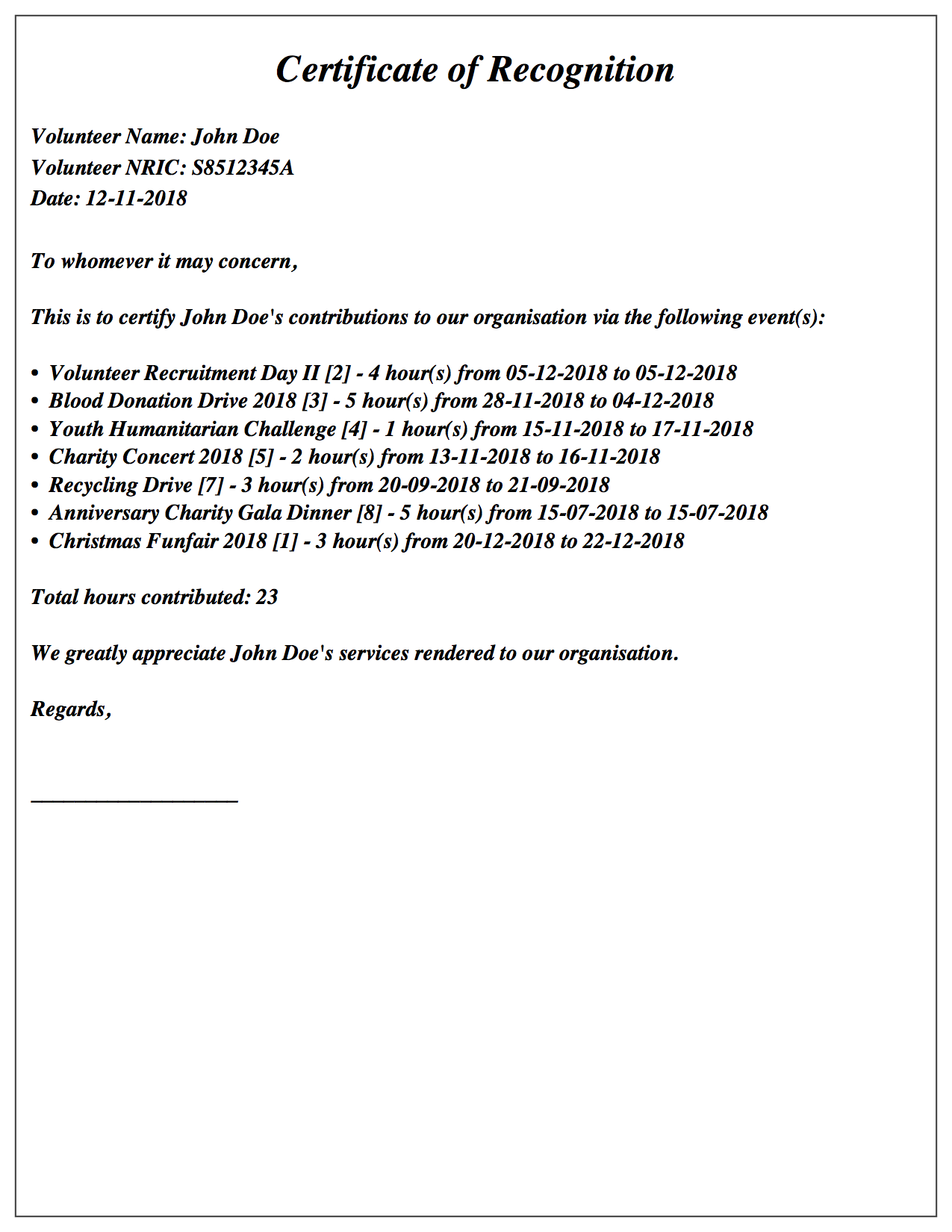
An empty line is provided at the end of the certificate for manual signing off. Feel free to add a signature or an organizational stamp to add credibility to the certificate!
To avoid exported file name clashes for volunteers with the same name, we have appended the volunteer’s NRIC to the filename as well. Exported file names will have the format '<VOLUNTEER NAME>_<VOLUNTEER NRIC>.pdf' (E.g. John Doe_S8512345A.pdf) |
Contributions to the Developer Guide
Given below is an excerpt from the section I contributed to the Developer Guide. It showcases my ability to write technical documentation which showcases the technical depth of my contributions to the project, along with my ability to critically analyse design considerations. |
Implementation
The following activity diagram shows us the control flow of the exportcert
feature. Analysing this diagram would be a good way to understand the intended functionality of this feature.
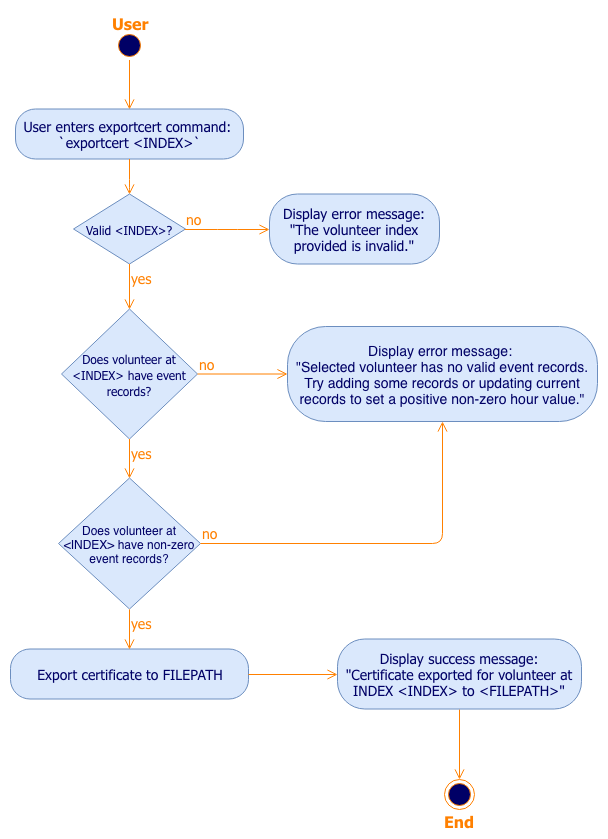
exportcert
command activity diagramThe following steps were involved in this feature’s implementation:
-
Support for accepting
exportcert
command.-
Added an ExportCertCommand class that extends Command.
-
Modified AddressBookParser class to accept an ExportCertCommand.
-
-
Support for accepting arguments as part of the command.
-
Modified ExportCertCommand class to take in an Index.
-
Added an ExportCertCommandParser class that parses the Index argument.
-
Modified the AddressBookParser to use the ExportCertCommandParser.
-
-
Retrieve the right volunteer based on the given Index.
-
Interact with the model to retrieve the filtered volunteer list.
-
Get the Volunteer at the specified Index.
-
-
Retrieve information on the events that this volunteer has been involved in, if any.
-
Interact with the model to get the filtered record list, and filter the record list further to find the records with the volunteer’s ID.
-
Retrieve the event IDs from the relevant filtered records, along with the hours contributed.
-
Get the Event that corresponds to the event ID, and retrieve its name, startDate and endDate for input into the certificate.
-
-
Use of Apache PDFBox to create and export a volunteer certificate with the information retrieved.
-
Involves the creation of a new PDDocument, with a PDPage to write the content to.
-
Writing of the information to a page content stream is then achieved using PDPageContentStream.
-
The following sequence diagrams show how the exportcert
operation will be executed for a valid command exportcert 1
. Analyse these diagrams to achieve a more detailed understanding of the internal interactions involved in the execution of this feature.
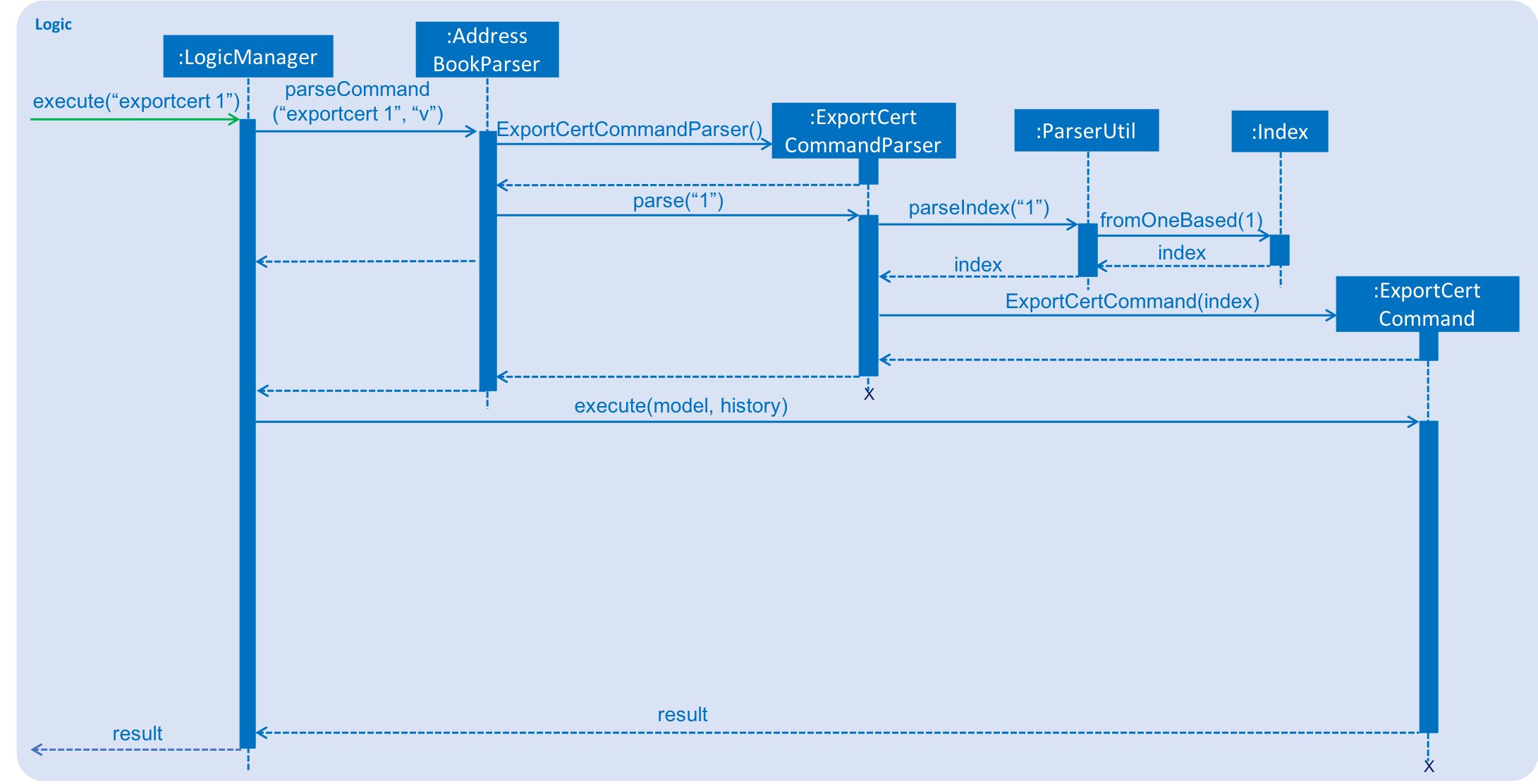
exportcert 1
with simplified ExportCertCommand executionThe next sequence diagram below looks at the ExportCertCommand’s execution in detail, including it’s interaction with the model.
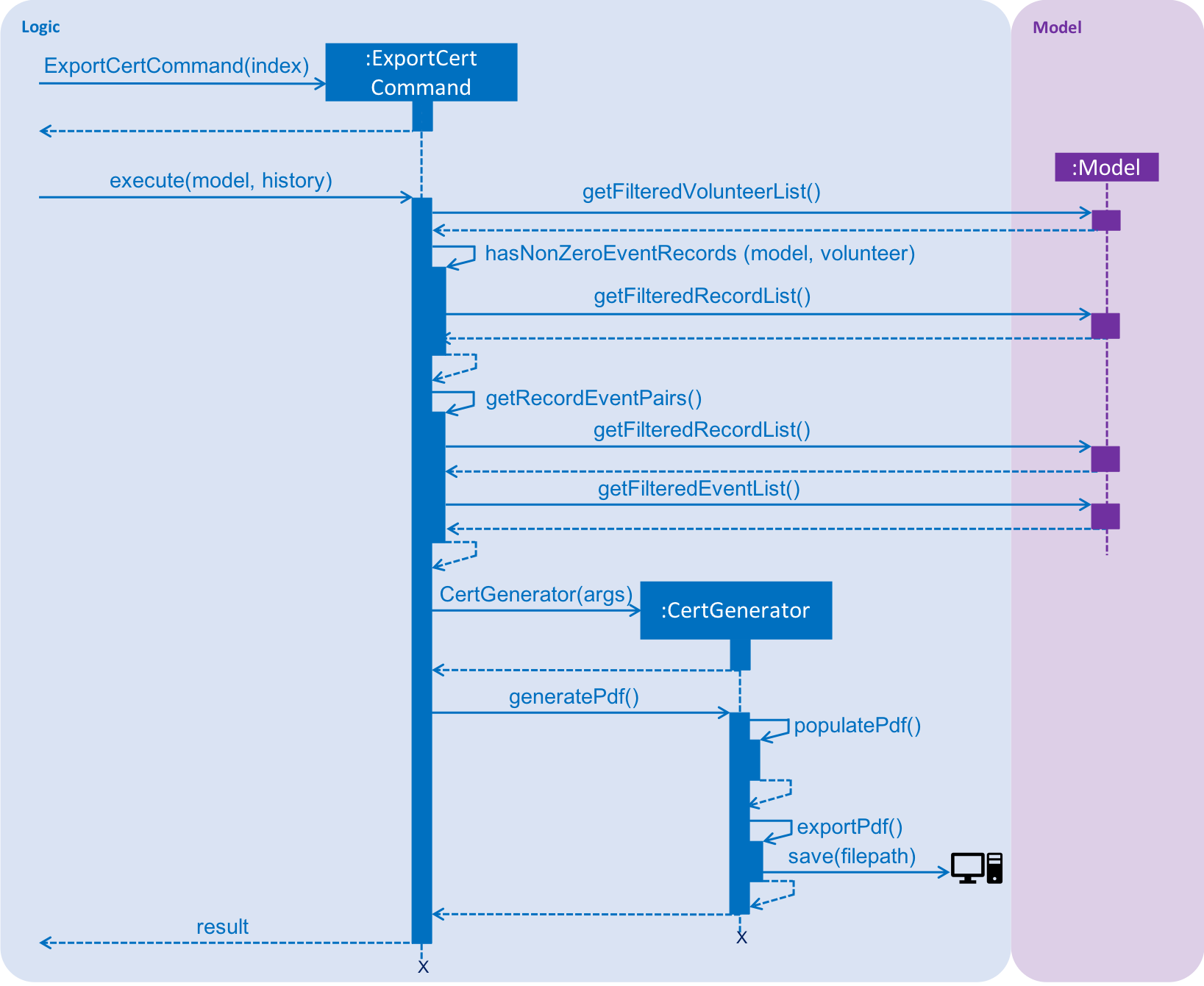
Design Considerations
Aspect: Medium of presentation
-
Alternative 1 (current choice): Export as PDF
Pros
Exports volunteer details in a convenient format for immediate use and distribution.
Cons
PDF template has to be preset within the application.
-
Alternative 2: Display volunteer data in a window within the application
Pros
Allows the volunteer manager flexibility as to what to do with the volunteer details. This could include manually inputting it into an existing certificate creation application, or a document template.
Cons
Requires more manual work on the volunteer manager’s side, especially when the process can be automated to enhance his/her productivity. Certificate templates are also infrequently updated, and thus the costs in terms of efficiency outweigh the benefits in terms of flexibility.
Aspect: Choice of PDF creation tool
-
Alternative 1 (current choice): Apache PDFBox
Pros
Open source, offers more specific functionality for PDFs than Apache FOP, and covers all of the pdf creation and manipulation functionality required for this feature.
Cons
Not the most efficient for creating PDFs (refer to this comparison study).
-
Alternative 2: Apache FOP
Pros
Open source, allows for conversion and formatting of XML data to PDF.
Cons
Resource intensive, not the most efficient for PDF creation, and lacks features such as updating and merging PDFs.
-
Alternative 3: iText
Pros
Fastest of the lot for PDF generation (refer to this comparison study).
Cons
Now only available as a free trial, and requires a license for extended use.
Aspect: Choice of export location
-
Alternative 1 (current choice): Export to the user’s current working directory
Pros
Putting the files relative to where the app is allows the user to locate, manage and access the exports easily as this is a portable app. The app jar and the exported files can be shifted to different locations together easily as well.
Cons
Navigating to this directory would be necessary if he/she wishes to access the files independent of using the application.
-
Alternative 2: Export to the user’s Desktop
Pros
Easy to access files when not using the application.
Cons
As it is a portable app, it may be cumbersome to keep navigating to the Desktop to access the exports when using the application. It also becomes harder to move the app jar and exports together from place to place.
-
Alternative 3: Allow the user to specify the export filepath as an argument
Pros
Allows for greater customisability, thus catering to each user’s unique needs.
Cons
Requires user to have accurate prior knowledge of the machine’s filepath format. Moreover, support for all possible OS filepath formats within the application may not be possible as well (e.g. custom OS filepath).
Aspect: Organisation of PDF generation code
-
Alternative 1 (current choice): Encapsulate within a
CertGenerator
class with exposed public method(s)Pros
Allows for separation of concerns as handling command result or exceptions & retrieving volunteer data is separated from the creation, population and export of the certificate. Also allows for future extension of the PDF generation feature (e.g. generating event reports) through adding a
ExportPdf
abstract class or interface withgeneratePdf
,populatePdf
andexportPdf
methods to be implemented.Cons
Introduces inherent coupling between
ExportCertCommand
andCertGenerator
classes as currently, onlyExportCertCommand
includes the certificate generation functionality. -
Alternative 2: Leave PDF generation code inside a method within the
ExportCertCommand
classPros
Allows the certificate generation code to reside exactly where it is used, as the certificate generation functionality is currently only used by
ExportCertCommand
.Cons
Causes a low level of cohesion by packaging different functionalities within
ExportCertCommand
. The functionality to export PDFs also remains unabstracted, and thus extending the functionality to create e.g. event report PDFs would require repetition of the PDF generation code.